7.8. Programming
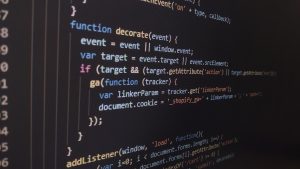
Software is created via programming, as discussed in Chapter 4. Programming is the process of creating a set of logical instructions for a digital device to follow using a programming language. The process of programming is sometimes called “coding” because the developer takes the design and encodes it into a programming language which then runs on the computer. A programming language is an artificial language that provides a way for a developer to create programming code to communicate logic in a format that can be executed by the computer hardware. Over the past few decades, many different types of programming languages have evolved to meet a variety of needs. One way to characterize programming languages is by their “generation.”
Generations of Programming Languages
Early languages were specific to the type of hardware that had to be programmed. Each type of computer hardware had a different low level programming language. In those early languages very specific instructions had to be entered line by line – a tedious process.
First generation languages were called machine code because programming was done in the format the machine/computer could read. So programming was done by directly setting actual ones and zeroes (the bits) in the program using binary code. Here is an example program that adds 1234 and 4321 using machine language:
10111001 00000000 11010010 10100001 00000100 00000000 10001001 00000000 00001110 10001011 00000000 00011110 00000000 00011110 00000000 00000010 10111001 00000000 11100001 00000011 00010000 11000011 10001001 10100011 00001110 00000100 00000010 00000000
Assembly language is the second generation language and uses English-like phrases rather than machine-code instructions, making it easier to program. An assembly language program must be run through an assembler, which converts it into machine code. Here is a sample program that adds 1234 and 4321 using assembly language.
MOV CX,1234 MOV DS:[0],CX MOV CX,4321 MOV AX,DS:[0] MOV BX,DS:[2] ADD AX,BX MOV DS:[4],AX
Third-generation languages are not specific to the type of hardware on which they run and are similar to spoken languages. Most third generation languages must be compiled. The developer writes the program in a form known generically as source code, then the compiler converts the source code into machine code, producing an executable file. Well-known third generation languages include BASIC, C, Python, and Java. Here is an example using BASIC:
A=1234 B=4321 C=A+B END
Fourth generation languages are a class of programming tools that enable fast application development using intuitive interfaces and environments. Many times a fourth generation language has a very specific purpose, such as database interaction or report-writing. These tools can be used by those with very little formal training in programming and allow for the quick development of applications and/or functionality. Examples of fourth-generation languages include: Clipper, FOCUS, SQL, and SPSS.
Why would anyone want to program in a lower level language when they require so much more work? The answer is similar to why some prefer to drive manual transmission vehicles instead of automatic transmission, namely, control and efficiency. Lower level languages, such as assembly language, are much more efficient and execute much more quickly. The developer has finer control over the hardware as well. Sometimes a combination of higher and lower level languages is mixed together to get the best of both worlds. The programmer can create the overall structure and interface using a higher level language but use lower level languages for the parts of the program that are used many times, require more precision, or need greater speed.
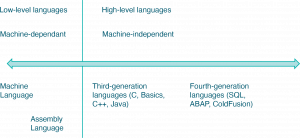
Programming Tools
To write a program, you need little more than a text editor and a good idea. However, to be productive you must be able to check the syntax of the code, and, in some cases, compile the code. To be more efficient at programming, additional tools, such as an Integrated Development Environment (IDE) or computer-aided software-engineering (CASE) tools can be used.
Integrated Development Environment
For most programming languages an Integrated Development Environment (IDE) can be used to develop the program. An IDE provides a variety of tools for the programmer, and usually includes:
- Editor. An editor is used for writing the program. Commands are automatically color coded by the IDE to identify command types. For example, a programming comment might appear in green and a programming statement might appear in black.
- Help system. A help system gives detailed documentation regarding the programming language.
- Compiler/Interpreter. The compiler/interpreter converts the programmer’s source code into machine language so it can be executed/run on the computer.
- Debugging tool. Debugging assists the developer in locating errors and finding solutions.
- Check-in/check-out mechanism. This tool allows teams of programmers to work simultaneously on a program without overwriting another programmer’s code.
Examples of IDEs include Microsoft’s Visual Studio and Oracle’s Eclipse. Visual Studio is the IDE for all of Microsoft’s programming languages, including Visual Basic, Visual C++, and Visual C#. Eclipse can be used for Java, C, C++, Perl, Python, R, and many other languages.
CASE Tools
While an IDE provides several tools to assist the programmer in writing the program, the code still must be written. Computer-Aided Software Engineering (CASE) tools allow a designer to develop software with little or no programming. Instead, the CASE tool writes the code for the designer. CASE tools come in many varieties. Their goal is to generate quality code based on input created by the designer.
“Chapter 10: Information Systems Development” from Information Systems for Business and Beyond (2019) by David Bourgeois is licensed under a Creative Commons Attribution-NonCommercial 4.0 International License, except where otherwise noted.