Programming on the Server Side
40 PHP Session Management
Learning Outcomes
When you have completed this chapter, you will be able to:
- Explain what it means to say that HTTP is a stateless protocol
- Use standard PHP session management techniques to maintain user state across multiple page loads and AJAX requests in a web app.
- Implement session management for user authentication in a PHP web app.
In the HTTP Parameters chapter, you were shown how to create simple, two-page PHP apps as shown in Figure 1 below. This figure shows the HTTP Request messaging between pages as well as a flow chart for the activities within each page that generate the HTML on the server.
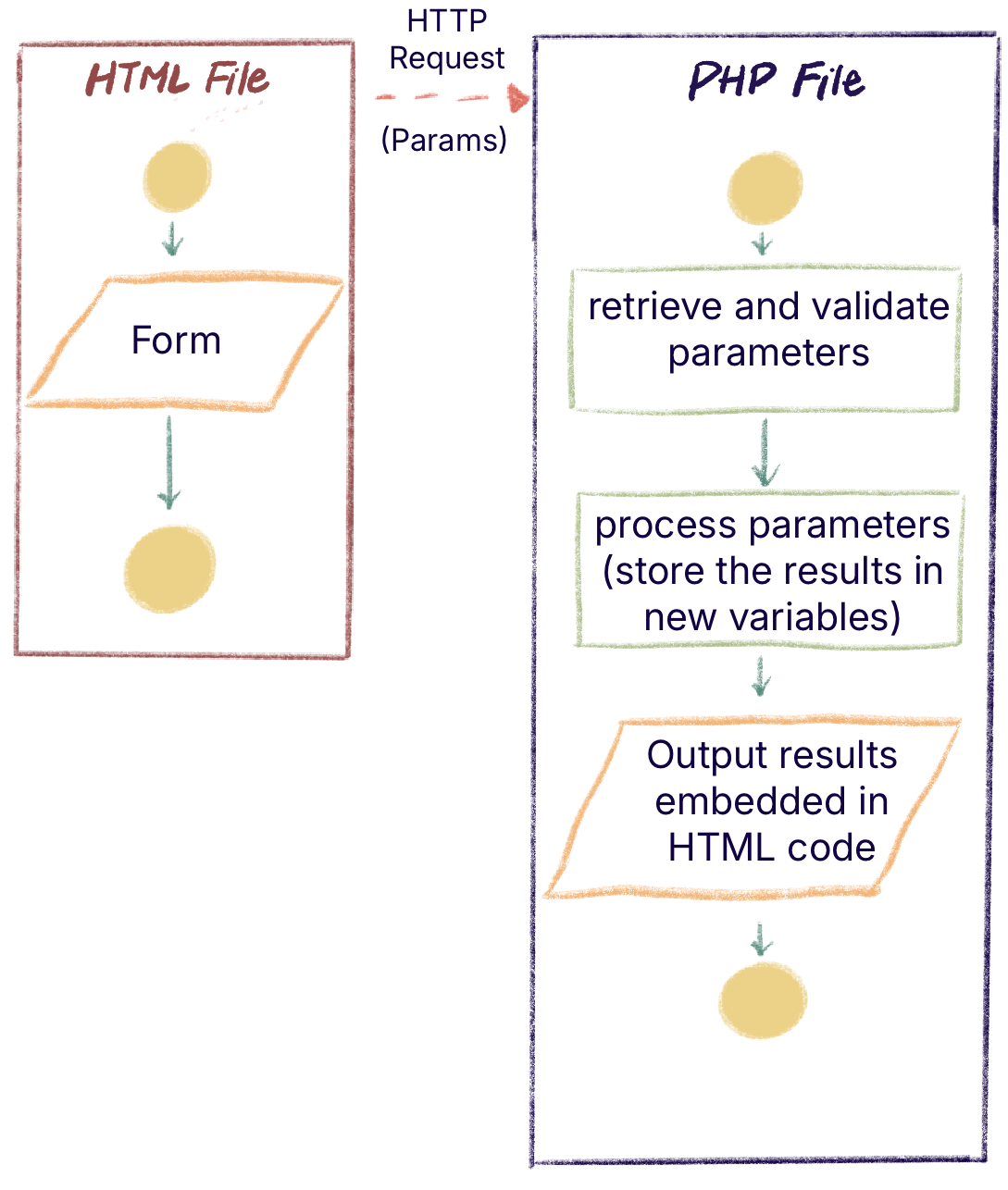
The first page in such an app is written in HTML and contains code to display a form with a form that redirects to a second page. The server simply outputs this code directly into the HTTP Response. The second page contains PHP code to process the parameters sent from the form and output the results into the HTTP Response.
The above setup works well for two-page apps, but not for an app with three or more pages. For example, the first page of your app might contain section 1 of a quiz, formatted as a form that the user submits to move on to the second page. Then the second page could contain section 2 of the quiz and the third page displays the results. This three-page situation is shown in Figure 2 below, with some question marks for work that needs to be filled in.
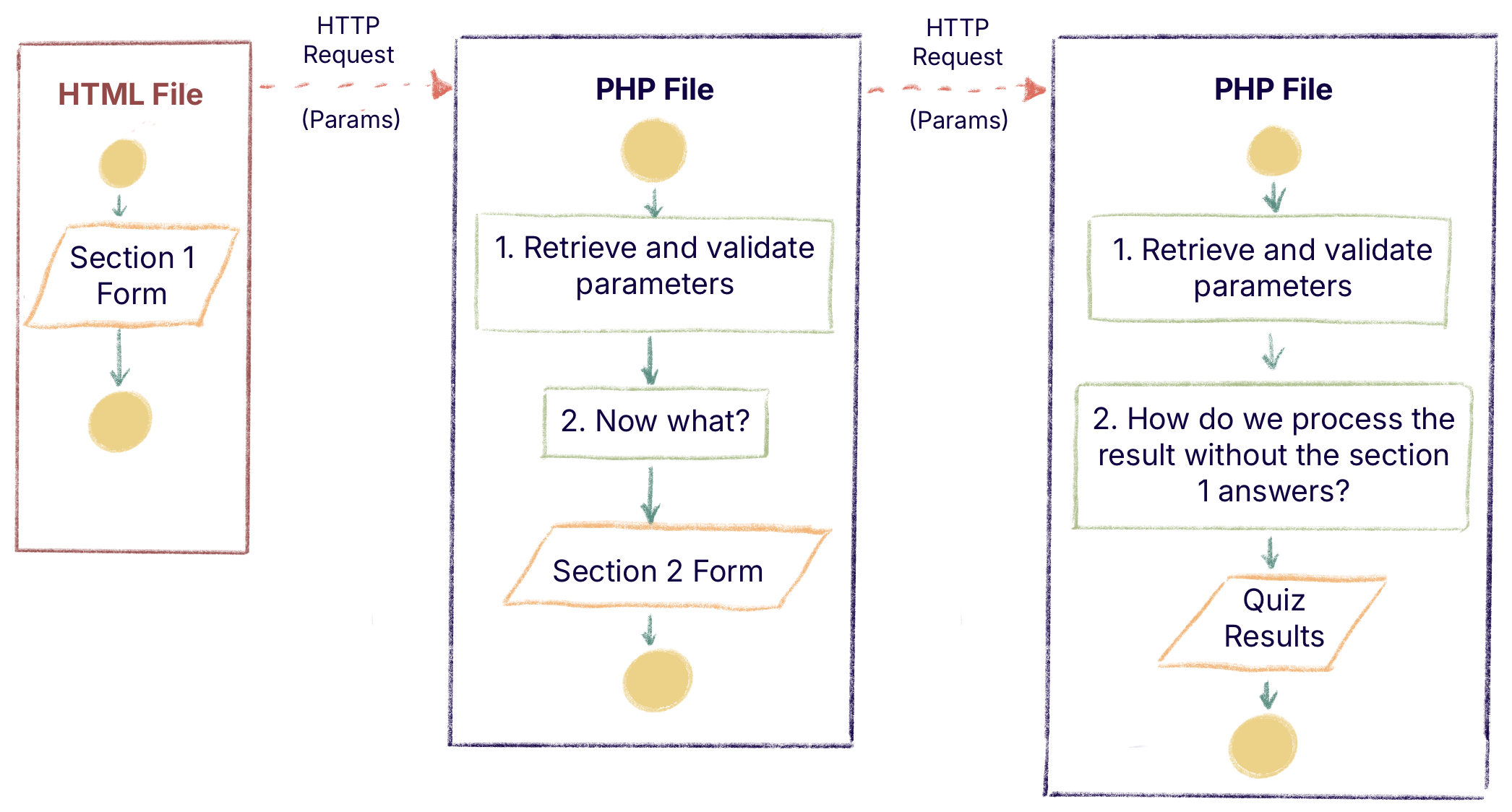
The problem posed by the structure above is that the third page must process information from parameters sent by both forms, but it only has direct access to the parameters from the second page. The parameters from page 1 were only sent to page 2. The app needs to remember everything that happened during this user’s entire, three page session with the server. This is the problem of Session Management.
Managing Session State
HTTP is a Stateless Protocol. This means there is no built-in mechanism for linking one HTTP Request with another. But most interactions between a user and a web app span more than one HTTP Request. For example, a user might be filling in a multi-part form, spread across different pages as depicted above. Or they might log in, search for products, fill a shopping cart, and head to the checkout, triggering 1 or more HTTP Requests at each step. These “conversations” between client and server are called sessions. At any given moment there might be dozens, hundreds, or thousands of clients, each in the middle of its own session with the server. Without special session management code, a web app cannot know upon receiving a new HTTP Request what session it belongs to and what state the conversation is in.
In the Client-side Storage chapter, we looked at how to use the localStorage and sessionStorage objects to perform a kind of session management on the client. This chapter is about session management that mostly takes place on the server side. In the PDO for SELECT chapter, we looked at how to use GET parameters and hidden input elements as a form of primitive session management that works in specific situations. This chapter is about a more general solution.
PHP Session Management
The easiest way to manage a session in PHP is to make use of the built-in session-management system. PHP implements session management by sending a cookie (a small piece of named data) to the client as part of the HTTP Response. The cookie is called PHPSESSID and it contains a unique id to identify the user. This cookie is stored in the browser, linked to the domain that sent it. Cookies can be set to expire or persist forever, but by default, PHPSESSID will expire when the browser is closed. When a user initiates a new HTTP request, the browser sends all cookies linked to that domain as part of the request. When a request arrives with a PHPSESSID cookie attached, a PHP script can retrieve, modify, and store information for the session using in a special associative array called $_SESSION. This process is illustrated in Figure 3 below.

Do It Yourself
This textbook is made using PressBooks, which is powered by PHP on the server. If you’re on Chrome, open the developer tools for this web page and see if you can find the PHPSESSID cookie in the Application tab. Can you read it’s value? If you find it, the value will be an unpronounceable string of digits and letters. This is your unique session ID on the eCampusOntario.pressbooks.pub server.
Can you figure out your session ID expiry date? What about the other cookies set by eCampusOntario.pressbooks.pub? When do they expire?
The Three-Page Quiz, Revisited
Now we are ready to look at a solution to the problem posed by the three-page web app. Figure 4 below shows a diagram of that solution, making use of PHP Session Management.
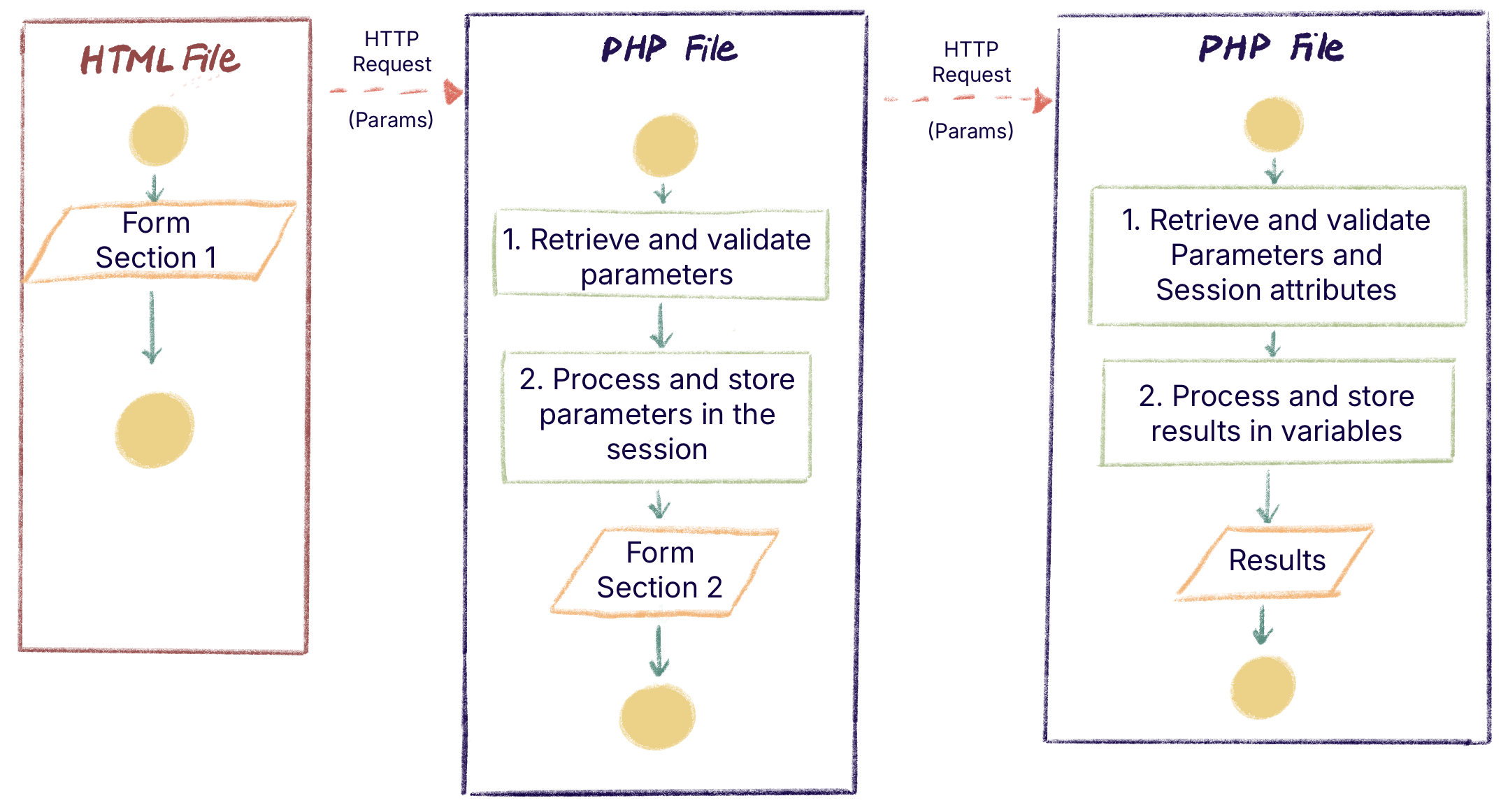
The first page contains a form that sends HTTP Parameters to the second page.
Then second page is a PHP script that does the following:
- Starts a session (causing the server to attach PHPSESSID to the HTTP Response)
- Retrieves the HTTP Request parameters
- Stores them in the session
- Displays the second form to send HTTP parameters to the third page
The third page is a PHP script that does the following:
- Accesses the existing session if it still exists (by default, existing sessions are deleted on the server after 30 minutes)
- Retrieves the HTTP Request parameters along with values stored in the session
- Processes the parameters and session information and stores the results in variables
- Displays the results
Session Management 101
The mechanics of PHP Session Management are fairly straightforward. The following functions and variables are available to any PHP script.
session_start();
- Starts or resumes a session.
- Must be called before any output has been written into the HTTP Response body (i.e., it must be called above the <!DOCTYPE html> line in a PHP web page). If you call this function after writing part of the response, it may still work, depending on how the Apache server happens to buffer its output in this instance. But you are risking an error each time the script is run.
<?php session_start(); ?> <!DOCTYPE html>
$_SESSION
- An associative array that stores data for the current session. You can store values of any type in this array, including objects and arrays. This variable is called a superglobal because you can access it inside functions and methods without having to declare it as a global variable.
isset($_SESSION["key"])
- The isset function can be used to check if a key exists in an associative array. It returns true if “key” has a value and false if it does not. You can use it to make sure the session is active and contains the information you need before attempting to use it. Otherwise, if the session has expired on the server or if the user has loaded this page directly without coming from another page of yours, you risk an undefined index error when you try to access the non-existent $_SESSION key.
session_destroy();
- Terminates the session and removes all data associated with it. The next call to session_start() will start a new session with an empty $_SESSION array. Note that you must call session_start() to resume the session first before you can destroy it.
Do It Yourself
Over on w3schools, there are some nice, simple session examples. You can run them over there if you like, or you can get the w3schools folder from the Full Stack Example Pack and run them via a server to see a slightly different version of the same examples, modified to fit the context of this textbook.
First try running the various files to see what they do. Then take a look at the code.
1_setSessionValues.php
- Starts/resumes a session and adds a “favcolor” and “favanimal” key to the $_SESSION array.
2_accessSessionValues.php
- Starts/resumes a session and displays the “favcolor” and “favanimal” entries, if they exist. Otherwise error.
3_modifySessionValues.php
- Starts/resumes a session and sets the “favcolor” key to a different value.
4_dumpSessionAndCookies.php
- Starts/resumes a session and uses var_dump to show the contents of $_SESSION and $_COOKIES.
($_COOKIES is a superglobal associative array that stores all the cookies that were sent along with the HTTP Request. It’s included here for your interest – you don’t need it for session management. But if you want to manage cookies yourself, you can do it with $_COOKIES and the setcookie() function. More on that over at w3schools.)
5_destroySession.php
- Starts/resumes a session and then destroys it.
Tracking the Conversation
Once a user finds your site, they may visit a number of pages, add things to shopping carts, save data for later, etc. If you want to track the state of the “conversation” the user is having with your site, you can store all the pertinent information in the $_SESSION array.
Do It Yourself
Get the conversation folder from the Full Stack Example Pack and run it via a server to see some basic session management in action. This is an example of a multi-step conversation with a user in which data is collected from a form at each step. It’s a a bit like a multi-stage registration process.
Start with step1.html and fill in each form before submitting. Watch the GET parameters in the URLs for each page. On the final page, the app reports all the information it collected on previous pages, then destroys the session.
Here’s a rundown of what is happening at each step. Check the code to make sure you understand.
step1.html
- Uses a form to get the user’s name and sends it as a GET parameter to step2.php.
step2.php
- Calls session_start() to create a new session. Stores the name parameter from step 1 in $_SESSION. Uses a form to get the user’s age and redirects to step3.php.
step3.php
- Calls session_start() to resume the session. Stores the age parameter from step 2 in $_SESSION. Uses a form to get the user’s favorite color and redirects to step4.php.
step4.php
- Calls session_start() to resume the session. Accesses and reports the user’s favorite color from the GET parameter. Accesses and reports the user’s name and age from $_SESSION. Destroys the session with session_destroy().
Predict what will happen if you reload step4.php after it has already displayed its output once. Try it and see if you are correct.
Authentication and Session Management
One important use of session management is to keep a user logged in after they have authenticated themselves. You can do that simply by adding a flag to the $_SESSION variable to indicate that they are logged in.
Do It Yourself
Get the login folder from the Full Stack Example Pack and run it via a server to see some basic session management for authentication. To login, the userid is “samscott” and the password is “samspassword”. Log in and visit the pages on the menu (here.php and there.php), then log out by visiting the logout.php page.
After you have logged out, try visiting here.php and there.php again, by typing the URLs into the address bar of the browser. What happens now?
This is a simple example of a site that won’t allow access to any pages if the user in the session is not authenticated. The username and password are hardcoded into the menu.php script.* If they are correct, the userid is stored in $_SESSION[“userid”]. If $_SESSION[“userid”] is set, then the current session is authorized to receive any page on the site. Otherwise, access is denied.
Here are a few more details:
index.html
- Uses a form to get the userid and password, sends it to menu.php.
menu.php
- If it gets POST parameters for userid and password, it checks them. If they are correct, it creates a $_SESSION value for the key “userid”. Then it either displays a menu or displays the message “access denied”. If the userid and password are no good, it also destroys the session.
here.php and there.php
- These scripts represent places to go within the web site. Each one checks $_SESSION[“userid”] and displays an error message instead of the regular page information if this key is not set.
logout.php
- Destroys the session and offers a link back to the login screen.
* Hardcoding a password is clearly not going to work for a site with multiple users. In that case, you would have a table of user information with a field for userid (probably the primary key) and another for password. See Exercise 6 in the Coding Practice section below for further hints on how to do that, and see the Data Security chapter sections on hashing and session storage for important security concerns related to logging in.
Session Management with Ajax
When you send an AJAX request to a PHP file, you can call session_start() and access the $_SESSION array just as you would on a PHP web page. If being logged in is required to access your app, then you should check to make sure a valid session exists by calling isset before you perform any tasks in response to an AJAX request. If there is no valid session, your script can echo an error code instead of its usual response.
Do It Yourself
Get the session_ajax folder from the Full Stack Example Pack and run it via a server to see some basic session management over AJAX. The app simulates a lineup by tracking the current session’s place in line. It starts each session at number 10 in line, then moves the customer up a random number of places in line each time they press the “Check Again” button.
Try out the app. When you reload, it will reset you to number 10 in line. Try running two sessions at once. You can do this by opening the app in Chrome, then opening it again using a Chrome incognito window, or with a different browser. With two sessions running, each should be at a different place in line.
Check the network activity. Now open the Network tab in developer tools to view the AJAX requests to checknum.php and the responses from the server.
HEre are a few more details.
index.php and js/takeanumber.js
- Sets a $_SESSION value representing how many customers are in front of the user in line (10 initially).
- Then the checknum.php script is requested via fetch whenever the button on the page is clicked.
checknum.php
- “Advances” the user in line by a random amount.
- Returns a JSON-encoded object to let the client know how many positions the user advanced, and what their position is now.
- When they get to position 0, the session is destroyed.
- If there is no active session, checknum.php returns the error code -1.
Take a look at the code to verify your understanding.
Check Your Understanding
Coding Practice
- The three-page quiz. Write the quiz app described at the beginning of this chapter. The first two pages should each contain three quiz questions requiring one-word text answers. Store the answers they enter in the session and then display their score on the final page. Don’t forget to destroy the session on that page as well.
- The three-page guessing game. Write a three page guessing game. On the first page, ask the user to enter a numeric range. On the second page, ask them to guess a random number in that range (generate and store this number in the session). Then on the third page, tell them whether they got the number right and destroy the session.
- Modify the guessing game app from the last exercise so that page 3 allows them to go back and try again. Don’t destroy the session until they get the number right.
- Modify all the pages of the conversation example from the Do it Yourself box above so that if any of the relevant session keys are not set, the user gets a nice looking page telling them their session has expired. Give them a link back to the first page. Research how to use JavaScript to automatically redirect them back to the first page in 10 seconds if they don’t click the link themselves.
- Modify the conversation example from the Do it Yourself box above so that the user information is stored in a single object or associative array which is stored in a session key called “user”. Create this object or associative array in step 2. It should have three fields/keys for name, age, and favorite color, initially filled out with default values.
- Create a user table in your database to store userid, password, age, and user type (an integer). Insert a few records. Then modify the login example from the Do it Yourself box above so that it connects to the database and issues a SELECT query for the user information using the given password and userid. If no rows are returned, the user is not logged in. If a row is returned, store all the user information except the password in the session and report it on menu.php, here.php, and there.php. (Storing passwords in plain text is not good security, but it will do for now. See the Data Security chapter for a better way.)
- AJAX guessing game. Create a one page guessing game called guess.php. When the user first arrives, generate a random number from 1 to 100 and store it in the session. Then give them a form to submit guesses. The guesses should be submitted to checkguess.php via AJAX and when the response comes back, the user should get an appropriate message. The script checkguess.php should return -1 if the guess is too low, 1 if it’s too high, 0 if it’s correct, -2 if the session is not found, and -3 if the parameters are not good. When the user guesses correctly, destroy the session.
- Slot Machine. In the Getting Started with PHP chapter, you may have created a simple slot machine which chose three fruits at random and paid out different amounts depending on whether there were two or three matches. Now expand it to send AJAX requests to spin the wheels and compute the payout to the user. This information should be sent back to the program in a JSON-encoded object or associative array. Return an error code if there is no active session. Keep track of the user’s winnings and allow them to bet different amounts on different spins. Give them 10 credits to start with and add to it or subtract from it as they play. Don’t let them bet less than 1 or more than they have left (have your PHP script return error codes in these cases). When they run out of money, it’s game over – kill the session.
Feedback/Errata