Programming on the Server Side
31 Getting Started with PHP
A Full Stack Web App is a collection of computer programs that run independently and communicate with each other to present the appearance of a single program. Web Apps are accessed through a browser (client) which retrieves files from a remote server. Some of the programs involved in the app run on the client, and others run on the server.
Figure 1 below (reproduced from the Client-server Architectures chapter) shows the client-server architecture of a typical web app.
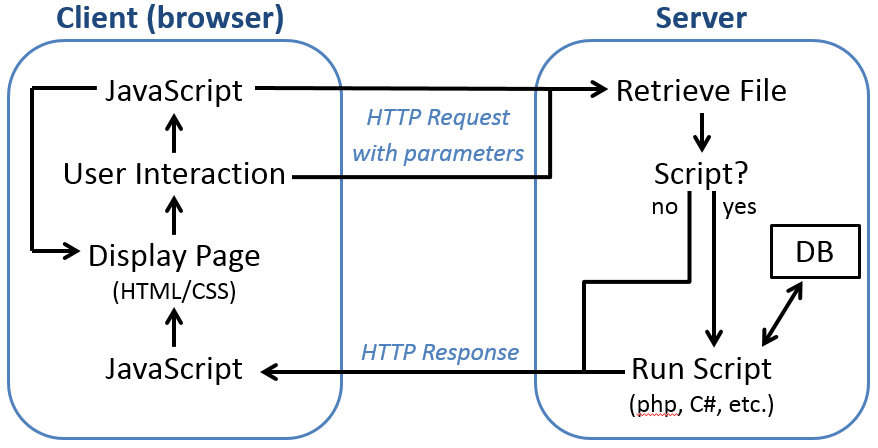
In this final section of the book, we will switch our attention from the client to the server. In terms of the 3-tiered architecture shown in Figure 2 below (also reproduced from the Client-server Architectures chapter) we’re moving our focus from the Presentation Tier of a web app to the Logic Tier where server-side scripts run, and the Data Tier where the database lives. We will use PHP programming language to write scripts for the Logic Tier, and we will retrieve and update the information in the Data Tier using the SQL query language.
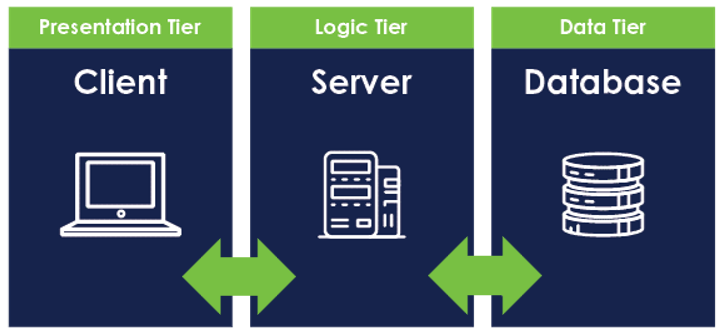
What are Server-side Scripts For?
Server-side scripts are run by a web server when it receives an HTTP Request. The job of a server-side script (in any language) is to help the server create the HTTP Response to send back to the user. It does this primarily by filling in custom content, often communicating with the Data Tier and drawing content from a database in the process.
You can write server-side scripts using PHP, JavaScript, Java, C#, or any other language. PHP was historically the first language used for server-side scripting and it was developed specifically for that purpose. (PHP was originally an acronym for Personal Home Page.) Despite the development of popular new frameworks for server-side development, PHP remains the most commonly found language on web servers around the world. This is partly due to the proliferation of WordPress sites like this one, because the WordPress software is written in PHP. But it’s also partly due to the ease ease and relatively low cost of obtaining, setting up, and managing a LAMP-like stack with an Apache web server, MySQL database management system, and PHP all bundled together. PHP remains a solid choice for small-scale, low traffic web apps.
Hello, World!
Let’s get started with the classic “Hello, World!” program. Unlike simple output programs in other languages, you will only be able to run the program by sending an HTTP Request to a web server. You can’t just double click a PHP file and run it in Chrome like you can for JavaScript. In what follows, it will be assumed that you’re running XAMPP for this (see Get Your Own Web Server for instructions) and that you will access your PHP scripts through http://localhost.
The basic PHP output statement is echo, but it does not send strings to a console or shell. Rather, the output of an echo statement ends up in the body of the HTTP Response that will be sent back to the browser by the web server. You can write a PHP web page mostly in plain HTML, embedding <?php ?> script tags where you want to run some code.
Do it Yourself
- Get template.php from the Full Stack Example Pack.
- Create a new folder named hello within XAMPP’s htdocs folder on your local machine. Copy template.php into that folder.
- Start up the XAMPP control panel, then use it to start the Apache server on your local machine.
- Use Chrome to navigate to http://localhost/hello. This causes an HTTP Request to be sent to the server running on your local machine. You should see a directory listing that includes template.php.
- Click template.php to run it. This launches a new HTTP Request.
- Open template.php using VS Code or your favorite code editor and add this line inside the <?php ?> tag:
<?php echo "<p>Hello, World!</p>"; ?>
- Reload template.php in Chrome to see the difference.
- Now change the name of the file to index.php and navigate to http://localhost/hello. This time, Apache will automatically run index.php instead of showing you a directory listing.
Use view page source in Chrome or use the Network tab in Chrome’s Developer Tools to view original text of the HTTP Response. This is the raw output of your program. The PHP interpreter executed the code inside the <?php ?> tag and placed the output of the code in that position. Note that, unlike with JavaScript, there is no PHP code sent from the server to the browser.
Server-side vs. Client Side Scripting
At first, this might feel a bit like client-side scripting, since you’re modifying the contents of a web page with code. But server-side scripting is quite different.
JavaScript code…
- is sent to the browser and executed on the browser,
- can be viewed by anyone who loads the web page, and
- can alter the browser’s internal model of the page (the DOM) in real time.
PHP code…
- is executed on the server and the output is sent to the browser,
- cannot be viewed through a browser (only the output can be viewed),
- can create different, customized, web pages for different users, but
- cannot make any changes to a page after it arrives at the browser.
Do it Yourself
Here are a few more things to try with the hello world program you wrote in the last Do it Yourself box. After each step, reload the page and take a look at the page source.
- Add more echo statements to the original <?php ?> tag, separated by semicolons.
- Add some HTML tags to the body of the document after the <?php ?> tag, then add more <?php ?> tags with echo statements.
- Make a CSS file and/or a JavaScript file and load it with a <link> or <script> tag.
You should also keep in mind that your PHP programs should return syntactically-correct HTML5 code. Remember to put all echo content inside HTML tags, and remember to close all your tags. The more you create HTML code inside echo statements, the harder it can be to visually inspect the HTML code for errors.
PHP Basics
You already know most of what you need to know about the PHP language because it is very similar in many respects to JavaScript.
PHP is an interpreted language that supports imperative programming (i.e. no classes or main function necessary).
PHP uses C-style syntax. This includes semicolons, braces (i.e., { and }), if, switch, while, for, do, operators, commenting, and so on. There are a few notable exceptions, though. The foreach loop is a little different in PHP (we’ll review it later), and there is an elseif keyword which you can use instead of else if. PHP also supports # for commenting, and you can use and and or if you prefer that to && and II.
PHP is dynamically-typed and weakly-typed and includes the === and !== Boolean operators to check both the type and the value of the operands. If you need a refresher on these concepts, you can review The JavaScript Type System. The PHP type system behaves in much the same way as JavaScript, though with a slightly different set of type conversion quirks.
Connections
The PHP language uses C-style syntax just like C, Java, C#, JavaScript and many other languages. But it also has a couple of syntactic features that are more Python-like.
Just like Python, PHP does not require variable declaration. PHP also supports Python-style # for commenting, and and and or (in addition to JavaScript-style &&, ||, and !). PHP also has an elseif keyword which functions just like Python’s elif keyword.
PHP Variables and Output
Variable names in PHP must start with a $ character, and are case sensitive. Variable declaration is not necessary. The scope of a variable is either global or local to a function, depending on where that variable is first assigned.
The statement below creates the variable $a and assigns an integer (int) value to it:
$a = 5;
PHP supports the following data types: int, float, bool, null, string, array, and object. PHP string literals can be single quoted or double quoted. A double-quoted string is richly interpreted, meaning that you can embed variable names and special codes such as \n into it. A single-quoted string is mostly uninterpreted and will print its contents verbatim.
Here are some example echo statements along with what they will print into the HTTP Response:
echo "The variable contains $a."; >>> The variable contains 5. echo 'The variable contains $a.'; >>> The variable contains $a.
Most of the time you can build output strings just using double-quotes and embedded variables. But if you want to use a concatenation operator to build strings, it’s the dot (.) operator. Don’t try to use the + operator for concatenation. That’s for arithmetic only in PHP.
Connections
PHP’s echo statement, variables, and richly-interpreted double-quoted strings are very similar to Bash, the language of the standard Linux shell. Bash also contains an echo statement, requires variable names to start with the $ character (though PHP requires this everywhere while Bash only requires it in certain contexts), and allows the embedding of variables into double-quoted strings.
Do it Yourself
Create a file called mario.php by copying template.php from the Full Stack Example Pack. Add the following tags to the body element (note the use of the built in rand function to generate a random integer):
<div class="player"> <?php $name = "Mario"; $health = 5.3; $health = $health - 0.5; $luckynum = rand(1, 10); echo "<h2>$name</h2>"; echo "<p>Life remaining: $health.</p>"; echo "<p>Lucky number: $luckynum </p>"; ?> </div>
Put mario.php it in your htdocs folder and load it through the browser to see the result. Did it work the way you expected? Look at the page source through the browser and make sure it makes sense to you. Then reload to see the lucky number change. Finally, try adding some CSS style rules to make it look a little better.
Gotcha: Concatenation
If you’re used to C-like languages or Python, at some point you’ll probably do something like this:
$name = "Ashkan"; echo "<p>Hello " + $name + "!</p>";
The above code will cause the string “0” to be placed into the HTTP Response. Why? (Hint: Weak typing).
Separation of Concerns
Sometimes it is easiest to write an entire page of HTML code and then just embed PHP variables or expressions into the output using the PHP expression tag <?= ?>. Here’s an example:
<p>The result is <?= $luckynum * 2 ?>.</p>
If the variable $luckynum holds the int value 7, the above will output the following string into the HTTP Response:
<p>The result is 14.</p>
The expression tag can help a little with maintaining a separation of concerns. You can put all your processing code at the top of the page in a <?php ?> tag, then use expression tags in the body section to display the results as necessary. Better yet, you can put large chunks of PHP code into a separate file and then load them through the use of include statements such as this one:
include "processing.php";
The include statement is like a copy and paste command. Any code in processing.php will execute as if it had been pasted into the location of the include statement.
Do it Yourself
Here’s another way to write the code from the last Do it Yourself box. This time we’re using the PHP expression tag and include statements to make the code a little neater.
Create a file called mario.php by copying template.php from the Full Stack Example Pack. At the very top of the file, put this <?php ?> tag:
<?php $name = "Mario"; $health = 5.3; $health = $health - 0.5; $luckynum = rand(1, 10); ?>
Then in the body element, instead of one big <?php tag, you can just write HTML and sprinkle in <?= ?> expression tags where necessary.
<div class="player"> <h2><?= $name?></h2> <p>Life remaining: <?= $health ?>.</p> <p>Lucky number: <?= $luckynum ?></p> </div>
Try loading the modified mario.php through a web server.
Now put the first <?php ?> tag into a separate file called vars.php. Then in mario.php, load the new file with an include statement, like this:
include "vars.php";
Try loading the modified mario.php through a web server again to make sure it still works.
Gotcha: Assignment vs. Comparison
Watch out for using = when you mean == or ===:
$guess = 5; if ($guess = 6) echo "<p>You guessed correctly!</p>";
As noted in The JavaScript Type System, $guess = 6 evaluates to the integer 6, which is converted to True for if statements.
Coding Practice
- Starting from template.php in the Full Stack Example Pack, write a PHP script that uses a for or a while loop to display all the even numbers from $start to $end in an unordered list (<ul>) where $start and $end are variables defined at the top of the script.
- Create a dice rolling PHP app. When the user loads the page, it should “roll” 5 dice with 6 sides each and display the result, formatted nicely. Each die roll should be in a separate HTML element (maybe a <div>). Include some CSS in an external style sheet to make it look good (i.e., it should look a bit like real dice). Use rand(1,6) to generate the random integers.
- Modify the program from question 2 so that any of the dice that roll a 1 are shown in red while the others are green. (Hint: create two classes and then use PHP to set the class name of each of the dice elements.)
- Write a PHP script that simulates a simple slot machine. When the page is loaded, the program should randomly choose three different fruit images to display for the user. If two of them match, they win something. If all three match they get the jackpot. You can use the fruit images from the images/fruit folder in the Full Stack Example Pack. Give it a little style to make it look a bit more like a slot machine. Include a button or link to reload the page so they can try again. (Hint: Use rand to generate random numbers, then use those numbers to create the src attribute for three img elements.)
- Create a PHP page that chooses a new temporary password and displays it on the page. The temporary password should be a random mix of upper- and lower-case letters and digits of length 16. (HINT: Use the rand function for random integers and the chr function to convert an ASCII code to a string. See http://www.asciitable.com/ for ASCII codes.)
- Write a PHP app that uses loops within loops to create a nicely formatted multiplication table in HTML. Include two variables at the top of the script that can be changed to alter the number of rows and columns displayed.
- You will have to research the use of the PHP date function for this. Write a PHP web app that displays the current time in an <h1> element using the format hh:mm:ss. If it’s night time, show it in dark color. If it’s daytime, show it in a bright color. Find a sun and a moon image online and display the appropriate image as well.